Class WScatterData
public class WScatterData extends WAbstractDataSeries3D
General information can be found at WAbstractDataSeries3D
. The model should be
structured as a table where every row represents a point. In the simplest case, there are three
columns representing the x-, y- and z-values. By default, this is column 0 for X, column 1 for Y
and column 2 for Z. It is also possible to provide an additional column containing information on
the color for each point. The same is possible for the size. Color-information in the model
should be present as a WColor
.
If these extra columns are not included, the MarkerBrushColorRole and MarkerScaleFactorRole can still be used to style individual points. These dataroles should be set on the values in the column containing the z-values.
The figure below shows an upward spiral of points, with droplines enabled and a pointsize of 5 pixels.
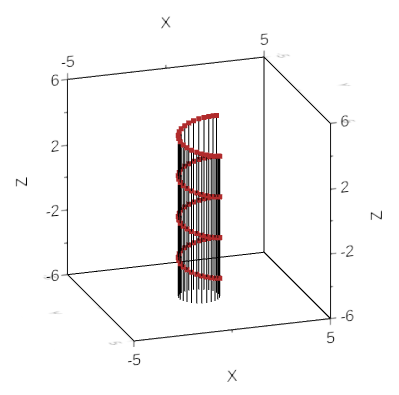
An example of WScatterData
-
Nested Class Summary
Nested classes/interfaces inherited from class eu.webtoolkit.jwt.WObject
WObject.FormData
-
Field Summary
Fields inherited from class eu.webtoolkit.jwt.chart.WAbstractDataSeries3D
chart_, model_, pointSprite_
-
Constructor Summary
Constructors Constructor Description WScatterData(WAbstractItemModel model)
Constructor. -
Method Summary
Modifier and Type Method Description void
deleteAllGLResources()
Delete GL resources.WPen
getDroplinesPen()
Returns the pen that is used to draw droplines.java.util.List<java.lang.Object>
getGlObjects()
void
initializeGL()
Initialize GL resources.boolean
isDroplinesEnabled()
Returns whether droplines are enabled.double
maximum(Axis axis)
Returns the computed maximum value of this dataseries along the given axis.double
minimum(Axis axis)
Returns the computed minimum value of this dataseries along the given axis.void
paintGL()
Update the client-side painting function.java.util.List<WPointSelection>
pickPoints(int x, int y, int radius)
Pick points on thisWScatterData
using a single pixel.java.util.List<WPointSelection>
pickPoints(int x1, int y1, int x2, int y2)
Pick points on thisWScatterData
inside of a rectangle.void
resizeGL()
Act on resize events.void
setColorColumn(int columnNumber)
void
setColorColumn(int columnNumber, int role)
void
setDroplinesEnabled()
Enables or disables droplines for all points.void
setDroplinesEnabled(boolean enabled)
Enables or disables droplines for all points.void
setDroplinesPen(WPen pen)
Sets the pen that is used to draw droplines.void
setSizeColumn(int columnNumber)
void
setSizeColumn(int columnNumber, int role)
void
setXSeriesColumn(int columnNumber)
Sets the column-index from the model that is used for the x-coordinate of all points.void
setYSeriesColumn(int columnNumber)
Sets the column-index from the model that is used for the y-coordinate of all points.void
setZSeriesColumn(int columnNumber)
Sets the column-index from the model that is used for the z-coordinate of all points.void
updateGL()
Update GL resources.int
XSeriesColumn()
Returns the column-index from the model that is used for the x-coordinate of all points.int
YSeriesColumn()
Returns the column-index from the model that is used for the y-coordinate of all points.int
ZSeriesColumn()
Returns the column-index from the model that is used for the z-coordinate of all points.Methods inherited from class eu.webtoolkit.jwt.chart.WAbstractDataSeries3D
getChart, getChartpaletteColor, getColorMap, getColorMapSide, getModel, getPointSize, getPointSprite, getPointSpriteTexture, getTitle, isColorMapVisible, isHidden, isLegendEnabled, loadPointSpriteTexture, setChart, setColorMap, setColorMapSide, setColorMapVisible, setColorMapVisible, setDefaultTitle, setHidden, setHidden, setLegendEnabled, setLegendEnabled, setModel, setPointSize, setPointSprite, setTitle
Methods inherited from class eu.webtoolkit.jwt.WObject
addChild, getId, getObjectName, remove, setFormData, setObjectName, tr
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
-
Constructor Details
-
WScatterData
Constructor.
-
-
Method Details
-
setDroplinesEnabled
public void setDroplinesEnabled(boolean enabled)Enables or disables droplines for all points.Enabling droplines will cause a line to be drawn from every point to the the ground-plane of the chart's plotcube. By default the droplines are disabled.
- See Also:
setDroplinesPen(WPen pen)
-
setDroplinesEnabled
public final void setDroplinesEnabled()Enables or disables droplines for all points. -
isDroplinesEnabled
public boolean isDroplinesEnabled()Returns whether droplines are enabled. -
setDroplinesPen
Sets the pen that is used to draw droplines.The default pen is a default constructed
WPen
.Note: only the width and color of the pen are used.
- See Also:
setDroplinesEnabled(boolean enabled)
-
getDroplinesPen
Returns the pen that is used to draw droplines. -
setXSeriesColumn
public void setXSeriesColumn(int columnNumber)Sets the column-index from the model that is used for the x-coordinate of all points.The default X column index is 0.
-
XSeriesColumn
public int XSeriesColumn()Returns the column-index from the model that is used for the x-coordinate of all points.- See Also:
setXSeriesColumn(int columnNumber)
-
setYSeriesColumn
public void setYSeriesColumn(int columnNumber)Sets the column-index from the model that is used for the y-coordinate of all points.The default X column index is 1.
-
YSeriesColumn
public int YSeriesColumn()Returns the column-index from the model that is used for the y-coordinate of all points.- See Also:
setYSeriesColumn(int columnNumber)
-
setZSeriesColumn
public void setZSeriesColumn(int columnNumber)Sets the column-index from the model that is used for the z-coordinate of all points.The default Z column index is 2.
Note that this column is also used to check for a MarkerBrushColorRole and a MarkerScaleFactorRole is no color-column or size-column are set.
-
ZSeriesColumn
public int ZSeriesColumn()Returns the column-index from the model that is used for the z-coordinate of all points.- See Also:
setZSeriesColumn(int columnNumber)
-
setColorColumn
public void setColorColumn(int columnNumber, int role) -
setColorColumn
public final void setColorColumn(int columnNumber) -
setSizeColumn
public void setSizeColumn(int columnNumber, int role) -
setSizeColumn
public final void setSizeColumn(int columnNumber) -
pickPoints
Pick points on thisWScatterData
using a single pixel.x,y are the screen coordinates of the pixel from the top left of the chart, and radius is the radius in pixels around that pixel. All points around the ray projected through the pixel within the given radius will be returned.
-
pickPoints
Pick points on thisWScatterData
inside of a rectangle.The screen coordinates (x1, y1) and (x2, y2) from the top left of the chart define a rectangle within which the points should be selected.
-
minimum
Description copied from class:WAbstractDataSeries3D
Returns the computed minimum value of this dataseries along the given axis.- Specified by:
minimum
in classWAbstractDataSeries3D
- See Also:
WAbstractDataSeries3D.maximum(Axis axis)
-
maximum
Description copied from class:WAbstractDataSeries3D
Returns the computed maximum value of this dataseries along the given axis.- Specified by:
maximum
in classWAbstractDataSeries3D
- See Also:
WAbstractDataSeries3D.minimum(Axis axis)
-
getGlObjects
public java.util.List<java.lang.Object> getGlObjects()- Overrides:
getGlObjects
in classWAbstractDataSeries3D
-
initializeGL
public void initializeGL()Description copied from class:WAbstractDataSeries3D
Initialize GL resources.This function is called by
initializeGL()
in the chart to which this dataseries was added.- Specified by:
initializeGL
in classWAbstractDataSeries3D
-
paintGL
public void paintGL()Description copied from class:WAbstractDataSeries3D
Update the client-side painting function.This function is called by
paintGL()
in the chart to which this dataseries was added.- Specified by:
paintGL
in classWAbstractDataSeries3D
-
updateGL
public void updateGL()Description copied from class:WAbstractDataSeries3D
Update GL resources.This function is called by
updateGL()
in the chart to which this dataseries was added. Before this function is called,deleteAllGLResources()
is called.- Specified by:
updateGL
in classWAbstractDataSeries3D
- See Also:
WAbstractDataSeries3D.deleteAllGLResources()
-
resizeGL
public void resizeGL()Description copied from class:WAbstractDataSeries3D
Act on resize events.This function is called by
resizeGL()
in the chart to which this dataseries was added.- Specified by:
resizeGL
in classWAbstractDataSeries3D
-
deleteAllGLResources
public void deleteAllGLResources()Description copied from class:WAbstractDataSeries3D
Delete GL resources.This function is called by
updateGL()
in the chart to which this dataseries was added.- Specified by:
deleteAllGLResources
in classWAbstractDataSeries3D
-