Class WPopupMenu
The menu implements a typical context menu, with support for submenu's. It is a
specialized WMenu
from which it inherits most of the API.
When initially created, the menu is invisible, until popup()
or exec() is called. Then, the menu will remain visible until an item is selected, or
the user cancels the menu (by hitting Escape or clicking elsewhere).
The implementation assumes availability of JavaScript to position the menu at the current mouse position and provide feed-back of the currently selected item.
As with WDialog
, there are two ways of using the menu. The simplest way is to use one
of the synchronous exec() methods, which starts a reentrant event loop and waits until the user
cancelled the popup menu (by hitting Escape or clicking elsewhere), or selected an item.
Alternatively, you can use one of the popup()
methods to
show the menu and listen to the triggered()
signal where you read
the getResult()
, or associate the menu with a button using WPushButton#setMenu()
.
You have several options to react to the selection of an item:
- Either you use the
WMenuItem
itself to identify the action, perhaps by specialization or simply by binding custom data usingWMenuItem#setData()
. - You can bind a separate method to each item's
WMenuItem.triggered()
signal.
Usage example:
// Create a menu with some items
WPopupMenu popup = new WPopupMenu();
popup.addItem("icons/item1.gif", "Item 1");
popup.addItem("Item 2").setCheckable(true);
popup.addItem("Item 3");
popup.addSeparator();
popup.addItem("Item 4");
popup.addSeparator();
popup.addItem("Item 5");
popup.addItem("Item 6");
popup.addSeparator();
WPopupMenu subMenu = new WPopupMenu();
subMenu.addItem("Sub Item 1");
subMenu.addItem("Sub Item 2");
popup.addMenu("Item 7", subMenu);
WMenuItem item = popup.exec(event);
if (item != null) {
// ... do associated action.
}
A snapshot of the WPopupMenu
:
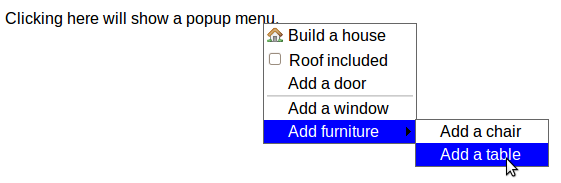
WPopupMenu example (default)
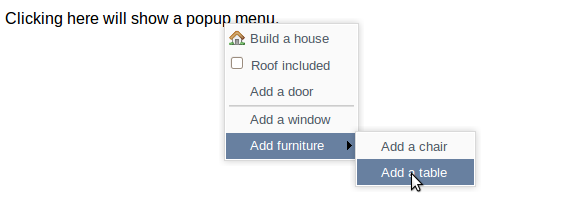
WPopupMenu example (polished)
- See Also:
-
Nested Class Summary
Nested classes/interfaces inherited from class eu.webtoolkit.jwt.WObject
WObject.FormData
-
Constructor Summary
ConstructorsConstructorDescriptionCreates a new popup menu.WPopupMenu
(WStackedWidget contentsStack) Creates a new popup menu.WPopupMenu
(WStackedWidget contentsStack, WContainerWidget parentContainer) Creates a new popup menu. -
Method Summary
Modifier and TypeMethodDescriptionSignal emitted when the popup is hidden.exec
(WMouseEvent e) Executes the the popup at the location of a mouse event.Executes the the popup at a position.final WMenuItem
Executes the popup besides a widget.exec
(WWidget location, Orientation orientation) Executes the popup besides a widget.Returns the last triggered menu item.boolean
Returns whether this popup menu should hide when an item is selected.void
popup
(WMouseEvent e) Shows the the popup at the location of a mouse event.void
Shows the the popup at a position.final void
Shows the popup besides a widget.void
popup
(WWidget location, Orientation orientation) Shows the popup besides a widget.void
remove()
Destructor.protected void
render
(EnumSet<RenderFlag> flags) Renders the widget.protected void
renderSelected
(WMenuItem item, boolean selected) final void
setAutoHide
(boolean enabled) Configure auto-hide when the mouse leaves the menu.void
setAutoHide
(boolean enabled, int autoHideDelay) Configure auto-hide when the mouse leaves the menu.void
setButton
(WInteractWidget button) protected void
setCurrent
(int index) void
setHidden
(boolean hidden, WAnimation animation) Hides or shows the widget.final void
Set whether this popup menu should hide when an item is selected.void
setHideOnSelect
(boolean enabled) Set whether this popup menu should hide when an item is selected.void
setMaximumSize
(WLength width, WLength height) Sets a maximum size.void
setMinimumSize
(WLength width, WLength height) Sets a minimum size.Signal emitted when an item is selected.Methods inherited from class eu.webtoolkit.jwt.WMenu
addItem, addItem, addItem, addItem, addItem, addItem, addItem, addMenu, addMenu, addSectionHeader, addSeparator, close, close, getContentsStack, getCount, getCurrentIndex, getCurrentItem, getInternalBasePath, getItems, getParentItem, getUl, indexOf, insertItem, insertItem, insertItem, insertItem, insertItem, insertItem, insertItem, insertMenu, insertMenu, isInternalPathEnabled, isItemDisabled, isItemDisabled, isItemHidden, isItemHidden, itemAt, itemClosed, itemSelected, itemSelectRendered, load, nextAfterHide, removeItem, select, select, setInternalBasePath, setInternalPathEnabled, setInternalPathEnabled, setItemDisabled, setItemDisabled, setItemHidden, setItemHidden
Methods inherited from class eu.webtoolkit.jwt.WCompositeWidget
addStyleClass, boxBorder, boxPadding, callJavaScriptMember, doJavaScript, enableAjax, find, findById, getAttributeValue, getBaseZIndex, getChildren, getClearSides, getDecorationStyle, getFloatSide, getHeight, getId, getImplementation, getJavaScriptMember, getLineHeight, getMargin, getMaximumHeight, getMaximumWidth, getMinimumHeight, getMinimumWidth, getObjectName, getOffset, getPositionScheme, getScrollVisibilityMargin, getStyleClass, getTabIndex, getTakeImplementation, getToolTip, getVerticalAlignment, getVerticalAlignmentLength, getWidth, hasFocus, hasStyleClass, isCanReceiveFocus, isDisabled, isEnabled, isHidden, isHiddenKeepsGeometry, isInline, isLoaded, isPopup, isScrollVisibilityEnabled, isScrollVisible, isSetFirstFocus, isThemeStyleEnabled, isVisible, propagateSetEnabled, propagateSetVisible, refresh, removeStyleClass, removeWidget, resize, scrollVisibilityChanged, setAttributeValue, setCanReceiveFocus, setClearSides, setDecorationStyle, setDeferredToolTip, setDisabled, setFloatSide, setFocus, setHiddenKeepsGeometry, setId, setImplementation, setInline, setJavaScriptMember, setLineHeight, setMargin, setObjectName, setOffsets, setPopup, setPositionScheme, setScrollVisibilityEnabled, setScrollVisibilityMargin, setSelectable, setStyleClass, setTabIndex, setThemeStyleEnabled, setToolTip, setVerticalAlignment
Methods inherited from class eu.webtoolkit.jwt.WWidget
acceptDrops, acceptDrops, addCssRule, addCssRule, addJSignal, addStyleClass, animateHide, animateShow, createJavaScript, disable, dropEvent, enable, getDropTouch, getJsRef, getParent, hide, htmlText, isExposed, isGlobalWidget, isLayoutSizeAware, isRendered, layoutSizeChanged, needsRerender, positionAt, positionAt, removeFromParent, removeStyleClass, render, resize, scheduleRender, scheduleRender, scheduleRender, setClearSides, setDeferredToolTip, setFocus, setHeight, setHidden, setLayoutSizeAware, setMargin, setMargin, setMargin, setMargin, setMargin, setOffsets, setOffsets, setOffsets, setOffsets, setOffsets, setToolTip, setVerticalAlignment, setWidth, show, stopAcceptDrops, toggleStyleClass, toggleStyleClass, tr
Methods inherited from class eu.webtoolkit.jwt.WObject
setFormData
-
Constructor Details
-
WPopupMenu
Creates a new popup menu.The menu is hidden, by default, and must be shown using
popup()
or exec(). -
WPopupMenu
public WPopupMenu()Creates a new popup menu. -
WPopupMenu
Creates a new popup menu.
-
-
Method Details
-
remove
public void remove()Description copied from class:WMenu
Destructor. -
popup
Shows the the popup at a position.Displays the popup at a point with document coordinates
point
. The positions intelligent, and will chose one of the four menu corners to correspond to this point so that the popup menu is completely visible within the window. -
popup
Shows the the popup at the location of a mouse event.This is a convenience method for
popup()
that uses the event's document coordinates.- See Also:
-
setButton
-
popup
Shows the popup besides a widget. -
popup
Shows the popup besides a widget. -
exec
Executes the the popup at a position.Displays the popup at a point with document coordinates
p
, usingpopup()
, and the waits until a menu item is selected, or the menu is cancelled.Returns the selected menu (or sub-menu) item, or
null
if the user cancelled the menu.- See Also:
-
exec
Executes the the popup at the location of a mouse event.This is a convenience method for
exec()
that uses the event's document coordinates.- See Also:
-
exec
Executes the popup besides a widget. -
exec
Executes the popup besides a widget. -
getResult
Returns the last triggered menu item.The result is
null
when the user cancelled the popup menu. -
setHidden
Description copied from class:WWidget
Hides or shows the widget.Hides or show the widget (including all its descendant widgets). When setting
hidden
=false
, this widget and all descendant widgets that are not hidden will be shown. A widget is only visible if it and all its ancestors in the widget tree are visible, which may be checked usingisVisible()
.- Overrides:
setHidden
in classWCompositeWidget
-
setMaximumSize
Description copied from class:WWidget
Sets a maximum size.Specifies a maximum size for this widget, setting CSS
max-width
andmax-height
properties.The default the maximum width and height are
WLength.Auto
, indicating no maximum size. ALengthUnit.Percentage
size should not be used, as this is (in virtually all cases) undefined behaviour.When the widget is a container widget that contains a layout manager, then setting a maximum size will have the effect of letting the size of the container to reflect the preferred size of the contents (rather than constraining the size of the children based on the size of the container), up to the specified maximum size.
- Overrides:
setMaximumSize
in classWCompositeWidget
- See Also:
-
setMinimumSize
Description copied from class:WWidget
Sets a minimum size.Specifies a minimum size for this widget, setting CSS
min-width
andmin-height
properties.The default minimum width and height is 0. The special value
WLength.Auto
indicates that the initial width is used as minimum size. ALengthUnit.Percentage
size should not be used, as this is (in virtually all cases) undefined behaviour.When the widget is inserted in a layout manager, then the minimum size will be taken into account.
- Overrides:
setMinimumSize
in classWCompositeWidget
- See Also:
-
aboutToHide
Signal emitted when the popup is hidden.Unlike the
WMenu.itemSelected()
signal,aboutToHide()
is only emitted by the toplevel popup menu (and not by submenus), and is also emitted when no item was selected.You can use
getResult()
to get the selected item, which may benull
.- See Also:
-
triggered
Signal emitted when an item is selected.Unlike the
WMenu.itemSelected()
signal,triggered()
is only emitted by the toplevel popup menu (and not by submenus).- See Also:
-
setAutoHide
public void setAutoHide(boolean enabled, int autoHideDelay) Configure auto-hide when the mouse leaves the menu.If
enabled
, The popup menu will be hidden when the mouse leaves the menu for longer thanautoHideDelay
(milliseconds). The popup menu result will be 0, as if the user cancelled.By default, this option is disabled.
-
setAutoHide
public final void setAutoHide(boolean enabled) Configure auto-hide when the mouse leaves the menu.Calls
setAutoHide(enabled, 0)
-
setHideOnSelect
public void setHideOnSelect(boolean enabled) Set whether this popup menu should hide when an item is selected.Defaults to true.
- See Also:
-
setHideOnSelect
public final void setHideOnSelect()Set whether this popup menu should hide when an item is selected.Calls
setHideOnSelect(true)
-
isHideOnSelect
public boolean isHideOnSelect()Returns whether this popup menu should hide when an item is selected.- See Also:
-
renderSelected
- Overrides:
renderSelected
in classWMenu
-
setCurrent
protected void setCurrent(int index) - Overrides:
setCurrent
in classWMenu
-
render
Description copied from class:WWidget
Renders the widget.This function renders the widget (or an update for the widget), after this has been scheduled using
scheduleRender()
.The default implementation will render the widget by serializing changes to JavaScript and HTML. You may want to reimplement this widget if you have been postponing some of the layout / rendering implementation until the latest moment possible. In that case you should make sure you call the base implementation however.
-